5 useful Typescript tips and tricks
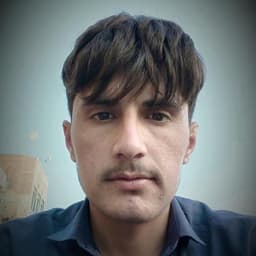
Khalid Kakar
Khalid simplifies complex modern web development topics into simple words with expertise in Next.js and React.js
In this artical we will be exploring 5 useful tips and tricks of the TypeScript and some of with React.JS.
1. React.ReactNode vs JSX.Element
The type of ReactNode infer that type which react render that can be number, string, undefiend, HTMLElement.
For example
import React from 'react'
const reactNode: React.ReactNode[] = [
'khalid',
undefiend,
123,
<div />
]
But in JSX.Element it only refer to htmlElements
For example
const jsxElement: JSX.Element[] = [
<div />,
<span />,
]
2. Generics
This is the best example of how generics can make the simple function far more useful.
// using any...
async function retry(
fn:()=>Promise<any>,
retries: number = 5
) {
try {
return await fn();
} catch (error) {
if(retries > 0){
console.log('Retrying..')
return await retry(fn, retries -1)
}
throw error
}
}
retry(()=>Promise.resolve('hello')).then((str)=>
// 2. resulting any
console.log(str)
)
For the same function using the generics
async function retry<T>(
fn:()=>Promise<T>,
retries: number = 5
) {
try {
return await fn();
} catch (error) {
if(retries > 0){
console.log('Retrying..')
return await retry(fn, retries -1)
}
throw error
}
}
retry(()=>Promise.resolve('hello')).then((str)=>
// 2. The type is infered
console.log(str)
)
as const
as const is most underrated feature it makes the types readonly that can be array, object and strings etc.
For example
// without as const
const routes = {
home: "/",
about:"/about",
service: "/service"
}
// can be changed
routes.home = '/whatever'
// with as const
const routes = {
home: "/",
about:"/about",
service: "/service"
} as const;
// can't be changed it warn us
routes.home = '/whatever'
const OPACITY = 0.5 as const;
4. ElementRef
ElementRef is veryful for finding the right type for the useRef in react.
import {useRef, ElementRef} from 'react';
const Comp = ()=>{
const divRef = useRef<ElementRef<'div'>>(null);
return (
<div ref={divRef}>
hello useRef
</div>
)
}
5. Destructing the types in typescript
This example explain the that how we can destructure the type in from object and arrays.
const roles = ['admin', 'superadmin', 'user']
// adapt if we add more roles to array
const Role1 = (typeof roles)[0 | 1 | 2];
// this works no matter how many roles we have in array
const Role2 = (typeof roles)[number]
HAPPY TYPES 😇